In the constantly evolving field of web development, prioritizing a seamless user experience remains of utmost importance. Users visit websites with specific goals, whether to find information, make a purchase, or access resources. The Angular search bar becomes essential in this case. Today, we’ll dive deep into the world of Angular search bars, exploring their significance, implementation, and how they can elevate your website’s functionality.
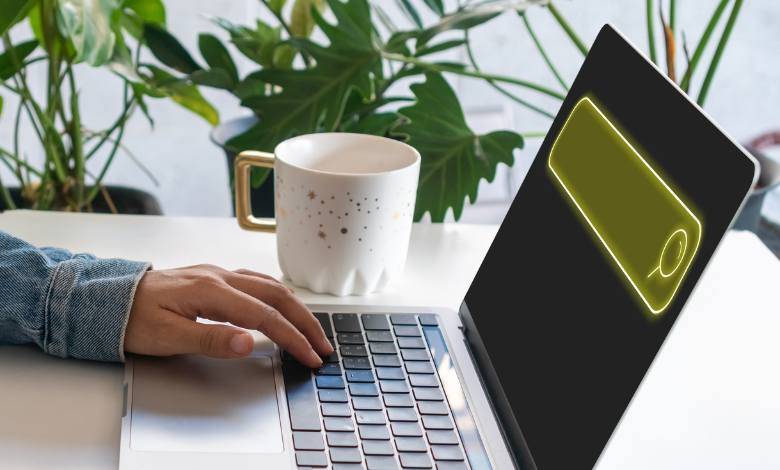
Table of Content
- Understanding Angular Search Bar
- Why is a Search Bar Crucial?
- The Angular Advantage
- Implementing an Angular Search Bar
- Enhancing the Search Experience
- SEO Benefits of Angular Search Bars
Understanding Angular Search Bar
At the heart of any effective website lies the ability for users to search and find what they need quickly. Angular, a widely used JavaScript framework, offers a powerful solution for seamlessly integrating a search bar into your web application. An Angular search bar, often referred to as an Angular search box or Angular search box, empowers your users to search through your website’s content effortlessly.
Why Angular?
Angular, a widely acclaimed JavaScript framework, has earned its reputation for its prowess in constructing dynamic and engaging web applications. When it comes to implementing a search bar on your website, Angular offers a range of advantages that make it an excellent choice.
Angular’s bidirectional data binding streamlines real-time search result updates as users input queries into the search bar. Consequently, users can instantly access pertinent results, obviating the need for page refreshes.
Additionally, Angular boasts an extensive arsenal of tools and libraries, which can significantly simplify the development workflow. Notably, the Angular Material library comes to the forefront, providing a repertoire of pre-designed components, including a fully functional search bar. This search bar can be seamlessly incorporated into your project, resulting in substantial time and effort savings.
Setting up your Angular app
To harness the power of Angular and implement an Angular search bar, you first need to set up your Angular application. This initial step is straightforward, so don’t worry if you haven’t already done so.
Angular provides a handy tool known as the Angular CLI (Command Line Interface). Your Angular project can be started with a single command. Here’s how:
ng new your-search-app
This command will generate a basic Angular project structure for you to work with.
Angular’s components — Making your app dynamic
Angular’s component-based architecture allows you to break your web application into reusable and manageable pieces. When implementing a search bar, you’ll typically create a dedicated component for it. This component will consist of HTML, TypeScript, and CSS files that define the search bar’s behavior and appearance.
To create a new component, you can use the Angular CLI:
ng generate component search-bar
Once your component is generated, you can customize it to meet your specific requirements. You can add HTML code for the search bar input field, apply styles using CSS, and write TypeScript code to handle user input and search functionality.
Angular Events — Interacting with the user
User interaction is at the heart of any search bar. Angular makes it easy to capture and respond to user events. You can use Angular event binding to listen for events like key presses and mouse clicks.
For example, you can use (keyup) event binding to detect when a user types in the search bar and trigger a search function:
<input type=”text” (keyup)=”search($event.target.value)”>
In this example, the search function will be called every time the user types in the input field, providing the search query as a parameter.
Search-specific Issues
While adding a search bar to your website can greatly enhance its usability, there are specific issues you should consider to ensure a smooth user experience.
Performance: As your website grows, the search functionality may need to handle a large dataset. To maintain performance, you should implement techniques like debouncing to reduce the number of search requests and optimize your backend for fast searches.
Mobile Responsiveness: Make certain that your Angular search bar adapts seamlessly to various screen sizes and device types. Angular’s flexibility and responsive design features can help you achieve this.
Accessibility: Including users with disabilities in the accessibility process is crucial. Make sure your search bar is keyboard navigable and complies with accessibility standards.
UI/UX Design: The design of your search bar should be user-friendly, with clear visual search cues and feedback. In addition to providing functional components, Angular Material also provides visually appealing components.
Why is a Search Bar Crucial?
Imagine landing on a website with a plethora of information, product searches, or service searches. Without a search bar, finding a specific item or piece of information can be akin to searching for a needle in a haystack. If users are frustrated, they may abandon your site, resulting in a poor user experience.
A search bar solves this problem by offering users a convenient way to access the content they seek. Whether you’re managing a blog, running an e-commerce platform, or maintaining a knowledge base, the integration of an Angular search bar holds the potential to greatly improve user satisfaction.
The Angular Advantage
Google’s Angular is a popular JavaScript framework known for its versatility and powerful features. When it comes to integrating a search bar into your website, Angular offers several advantages:
Component-Based Architecture: Angular’s component-based architecture makes it easy to create reusable search bar components that can be added to multiple pages of your website.
Real-Time Updates: Angular’s two-way data binding ensures that search results are updated in real-time as users type their queries, providing a dynamic and responsive search experience.
Integration with Angular Material: Angular Material is a design framework that offers pre-built UI components, including a search bar component. You can seamlessly integrate Angular Material’s search bar into your application for a polished and consistent look.
Implementing an Angular Search Bar
Now that we understand the importance of an Angular search bar, let’s explore how to implement one. Here is a step-by-step breakdown of the process.
Step 1: Set Up Your Angular Project
If you haven’t already, you’ll need to set up an Angular project. With the CLI (Command Line Interface) for Angular, you can accomplish this. Once your project is set up, you can proceed to the next steps.
Step 2: Install Angular Material
Angular Material provides a set of UI components, including the search bar, that can be easily integrated into your Angular project. The following command should be run in your project directory to install Angular Material:
ng add @angular/material
Choosing the right theme and typography settings is as easy as following the prompts.
Step 3: Create the Search Bar Component
Now, let’s create a dedicated search bar component for your application. Use the Angular CLI to generate a new component:
ng generate component search-bar
Your search bar component will be created in a folder that you can access by running this command.
Step 4: Implement the Search Functionality
In the search-bar.component.html file, you can add the Angular Material search bar component. Here’s an example:
<mat-form-field class=”example-form-field”>
<input matInput (keyup)=”search($event.target.value)” placeholder=”Search”>
<button mat-button *ngIf=”query” (click)=”clearSearch()”>Clear</button>
</mat-form-field>
In the above code, we’ve created a search input field that triggers the search function whenever the user types. The clearSearch function allows users to clear their search query.
Step 5: Implement the Search Logic
In your search-bar.component.ts file, you’ll need to implement the logic for handling search queries and displaying results. Here’s a basic example:
import { Component } from ‘@angular/core’;
@Component({
selector: ‘app-search-bar’,
templateUrl: ‘./search-bar.component.html’,
styleUrls: [‘./search-bar.component.css’]
})
export class SearchBarComponent {
query: string = ”;
search(query: string) {
// Implement your search logic here
}
clearSearch() {
this.query = ”;
}
}
In the search function, you can define how your application should handle search queries. This might involve making API calls to fetch search results or filtering data from your application.
Step 6: Style Your Search Bar
CSS styles can be applied to search-bar.component.css to customize your search bar’s appearance. Angular Material also provides theming options to match your application’s design.
Step 7: Add the Search Bar to Your Pages
Finally, you can add your newly created search bar component to any page in your Angular application by including the selector <app-search-bar></app-search-bar> in the respective HTML file.
Enhancing the Search Experience
To create an exceptional search experience for your users, consider the following tips:
1. Autocomplete Suggestions
Implementing autocomplete suggestions as users type can help them find what they’re looking for faster. Angular provides libraries like ng-bootstrap and ngx-bootstrap that offer autocomplete search components.
2. Filter and Sort Options
Enhance user experience by offering filter and sorting capabilities, allowing users to fine-tune search results. In e-commerce, users can filter products by price, category, or brand.
3. Error Handling
Handle errors gracefully by providing clear messages when no search results are found or when there’s an issue with the search functionality. This prevents user frustration.
4. Mobile Responsiveness
Ensure that your Angular search bar is responsive on all devices, including mobile phones and tablets. Angular Material’s components are mobile-friendly by default.
SEO Benefits of Angular Search Bars
Apart from improving the user experience, Angular search bars can also boost your website’s search engine optimization (SEO) efforts. Search engines like Google value websites that provide a user-friendly experience, and having a search bar can contribute to this. Here’s how:
1. Increased User Engagement
A user who effortlessly locates their desired content is more inclined to linger on your site, delve into various pages, and interact with your material. This heightened user engagement conveys favorable indicators to search engines, signifying the value of your website to users.
2. Reduced Bounce Rate
As users swiftly access the information they seek via a search bar, the likelihood of them swiftly returning to search engine database results dwindles. A lower bounce rate is a positive SEO signal, suggesting that your website meets users’ expectations.
3. Improved Content Visibility
As users search for specific keywords using your Angular search bar, they are essentially telling you what topics or products are of interest to them. This information can shape your content strategy, enabling you to generate content that is more precise and pertinent, aligning perfectly with user inquiries.
4. Enhanced Internal Linking
Search bars often provide links to relevant pages or products within your website. Incorporating internal links can enhance the distribution of PageRank, which serves as a gauge of a page’s significance, thereby positively impacting your overall SEO performance.
A Search Library: PartsLogic
To simplify the process of adding an Angular search bar to your website, you can leverage existing libraries and tools. One such library is PartsLogic, a powerful search library that is specifically designed for Angular applications.
PartsLogic offers a collection of Angular components and services that seamlessly integrate into your project. With PartsLogic, you can implement advanced search features like autocomplete, faceted search, and real-time filtering without the need for extensive custom coding.
In conclusion, the journey of incorporating an Angular search bar into your website is an exciting one. Angular’s prowess in creating dynamic and interactive web applications, coupled with its event-handling capabilities, makes it a natural choice for this task. However, don’t forget to address performance, responsiveness, accessibility, and user interface design concerns.
And if you’re seeking a shortcut to search success, consider enlisting the help of libraries like PartsLogic. With the right tools and know-how, you can take your website’s search functionality to new heights, providing your users with an experience they won’t forget. So, why wait? It’s time to embark on your Angular search bar adventure and redefine the way users explore your website.