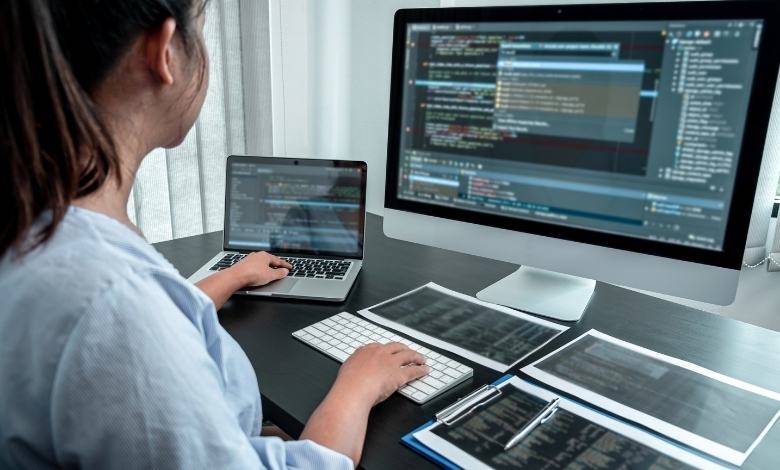
Table of Content
- Backend-Driven Autocomplete with an Internal API
- Adding a Query Suggestions Database
- Federated Search: Querying Multiple Dynamic Data Sources
- Building a Robust Search System Beyond Autocomplete
You’ve probably encountered autocomplete searches before, where users receive suggested searches as they type. This not only saves keystrokes and time for your users but can also reveal potential results they might otherwise have missed.
For instance, users will receive a variety of suggestions as they type “apple” into their product catalog search. You’ll need to gather potential matches from an API, database, or list of known terms in order to provide these matches.
Before delving into the process of creating your own autocomplete solution, let’s explore a robust, production-ready alternative:
We have previously discussed an autocomplete solution that elevates the simple drop-down menu into a rich and multi-faceted interactive user experience. Modern users expect more than just suggested queries when they begin typing a query. They desire access to a diverse range of data sources and results, along with multiple filters, categories, and images accompanied by helpful and highlighted text.
This open-source autocomplete library is fully customizable and adaptable to any industry or UI/UX design.
In this article, we’ll take a step back and guide you through building a basic autocomplete feature using JavaScript. As a result, you will gain an understanding of what makes up our production-level version. Furthermore, we’ll demonstrate how using additional front-end and back-end code in your autocomplete search will help you fine-tune it effectively.
To begin, let’s develop a proof-of-concept autocomplete demonstration. Our objective is to enable users to initiate text input in a search box and observe matching terms appearing beneath the search form, offering autocomplete suggestions as they type.
For any JavaScript autocomplete search functionality, the following components are essential:
- HTML code for the search form.
- CSS styling to format and display the search results.
- A data source for the autocomplete results.
- JavaScript for handling the autocomplete behavior.
Since we are starting with a basic implementation, we will retrieve exact match results from a predefined set of search terms. In this example, we’ll simulate an online store scenario where users are searching for specific items. If what they enter in the search box matches an item, the website will suggest that item.
Let’s begin with the HTML structure for the search box:
<form autocomplete=”off”>
<input type=”text” name=”q” id=”q” onKeyUp=”showResults(this.value)” />
<div id=”result”></div>
</form>
Disabling autocomplete (autocomplete=”off”) may seem unconventional, but it is necessary to prevent the browser’s automatic suggestions, which can interfere with our custom autocomplete.
A couple of important points to note are the use of the onKeyUp attribute, which triggers our JavaScript function, and the utilization of an empty div element to display the search results.
Next, here’s the JavaScript code responsible for receiving the search value, matching it against the predefined terms, and displaying suggestions:
var search_terms = [‘apple’, ‘apple watch’, ‘apple macbook’, ‘apple macbook pro’, ‘iphone’, ‘iphone 12’];
function autocompleteMatch(input) {
if (input == ”) {
return [];
}
var reg = new RegExp(input);
return search_terms.filter(function (term) {
if (term.match(reg)) {
return term;
}
});
}
function showResults(val) {
res = document.getElementById(“result”);
res.innerHTML = ”;
let list = ”;
let terms = autocompleteMatch(val);
for (i = 0; i < terms.length; i++) {
list += ‘<li>’ + terms[i] + ‘</li>’;
}
res.innerHTML = ‘<ul>’ + list + ‘</ul>’;
}
The search terms are stored in a simple JavaScript array at the top of the code. The browser calls the show results function after each keystroke, passing the current search query to the autocompleteMatch function to obtain a list of matching terms. Finally, the matched terms are added to the div element as an unordered list.
The matching terms will appear below the search box as bullet points when you run this code. However, the user experience can be enhanced. To achieve a better visual appearance, you can apply some CSS styles, which can be customized to match your website’s design:
#result {
border: 1px dotted #ccc;
padding: 3px;
}
#result ul {
list-style-type: none;
padding: 0;
margin: 0;
}
#result ul li {
padding: 5px 0;
}
#result ul li: hover {
background: #eee;
}
Although this is a basic implementation of autocomplete with JavaScript, it is still far from being suitable for production use. One significant limitation is that the data is sourced from a static array hardcoded within our JavaScript. To make this example more realistic, we should take a step toward incorporating a backend search request.
Backend-Driven Autocomplete with an Internal API
The basic JavaScript autocomplete example provides a foundation for the expected interaction patterns users anticipate. While there are always opportunities for design and interaction improvements, the core concept remains the same: as users input text into a search bar, they should see relevant potential searches or results displayed below. However, the crucial difference, which we will explore in the rest of this post, lies in how we retrieve and populate those results.
There is generally no finite list of possible queries available in real-world scenarios. The static implementation in the first section is only intended for demonstration purposes and isn’t suitable for production. To provide more dynamic and relevant results, you’ll need to send the user’s search query to a backend server, which handles the query and returns potential matches. This typically involves implementing an internal API to handle the search.
We can update the show results function from the previous section to something like this:
function showResults(val) {
const res = document.getElementById(“result”);
res.innerHTML = ”;
if (val == ”) {
return;
}
let list = ”;
fetch(‘/suggest?q=’ + val)
.then(function (response) {
return response.json();
})
.then(function (data) {
for (let i = 0; i < data.length; i++) {
list += ‘<li>’ + data[i] + ‘</li>’;
}
res.innerHTML = ‘<ul>’ + list + ‘</ul>’;
return true;
})
.catch(function (err) {
console.warn(‘Something went wrong.’, err);
return false;
});
}
Instead of using the autocompleteMatch function to compare the current query to potential queries, the local API endpoint /suggest is called. This endpoint returns a JSON array of potential matches.
To test this functionality, you can set up your own local API using a backend framework like Node.js or any other backend language. For example, the following Express route provides suggestions based on the q query parameter:
const express = require(‘express’);
const router = express.Router();
const data = [“apple”, “apple watch”, “iphone”, “iphone x”, “apple macbook”, “apple macbook air”, “apple macbook air pro 13”];
router.get(‘/suggest’, function (req, res, next) {
res.setHeader(‘Content-Type’, ‘application/json’);
res.end(JSON.stringify(data.filter(value => value.includes(req.query.q))));
});
module.exports = router;
Please note that if you deploy this to a production server, there may be a performance impact. Each lookup happens after a single key press, which might not be efficient when users type multiple keystrokes rapidly. To mitigate this, consider adding a delay on the frontend before making requests to the backend over the Internet. Techniques like network throttling can help improve the user experience.
Adding a Query Suggestions Database
The heart and soul of an autocomplete feature lie in its query suggestions database. This is where the system predicts what the user is likely to search for based on their input. Let’s dive deeper into this crucial component.
The Importance of a Robust Database
The quality and accuracy of suggestions depend on the database’s content. For example, a search engine’s autocomplete database would include popular search queries, trending topics, and user-specific history to provide personalized suggestions.
In e-commerce, the database would store product names, categories, and attributes. If the user types ‘smartphone,’ the database should offer suggestions like ‘iPhone 13 Pro Max’ or ‘Samsung Galaxy S21 Ultra.’
Populating the Database
To create an effective autocomplete database, you need to continuously collect and update relevant data. This can be achieved through web scraping, user interactions, or by integrating with external APIs.
For instance, an e-commerce website search might periodically scrape product data from manufacturers’ websites to ensure that the autocomplete suggestions stay up-to-date with the latest offerings.
Optimizing the Search Algorithm
The search algorithm used to query the database is critical. A real-time suggestion system should offer relevant suggestions quickly, efficiently, and in real-time. If your application requires a specific algorithm, such as sorting or fuzzy searching, you can choose from several options.
For instance, if you’re building a music streaming platform, you’d want your autocomplete to be capable of handling typos and suggesting the correct artist or song despite minor misspellings.
Federated Search: Querying Multiple Dynamic Data Sources
Autocomplete JavaScript becomes even more powerful when you need to query multiple dynamic data sources simultaneously. This scenario, known as federated search, is common in applications that aggregate information from various locations. Let’s dive into how autocomplete can handle this complex task.
Federated Search Challenges
The federated search involves querying multiple data sources, which can be databases, APIs, or external websites. Each source may have its own structure, data format, and response time. Here are some challenges that developers face when implementing federated search with autocomplete:
1. Data Integration
- Integrating data from diverse sources into a single autocomplete list can be complex. It requires mapping different data formats to a unified structure.
2. Response Time
- Some data sources may respond slower than others. Balancing the response time for a seamless user experience is crucial.
3. Data Freshness
- Ensuring that the autocomplete suggestions reflect the most up-to-date information from all sources can be challenging.
Autocomplete for Federated Search
To tackle these challenges, developers can implement autocomplete with federated search capabilities:
1. Multi-Source Query
- Develop a backend logic that can simultaneously query multiple data sources based on the user’s input. This may involve making asynchronous requests to various APIs or databases.
2. Data Normalization
- Normalize the data retrieved from different sources to create a consistent autocomplete suggestion format. This ensures that users see a coherent list of suggestions.
3. Response Prioritization
- Implement a prioritization mechanism to rank autocomplete suggestions based on relevance and the speed of data source responses. This ensures that the most pertinent suggestions appear at the top of the list.
4. Caching and Refresh
- Employ caching strategies to store frequently queried data temporarily. Refresh the cache periodically to maintain data freshness.
5. User Feedback
- Over time, incorporate mechanisms that allow users to provide feedback so that suggestions become more accurate. Users can indicate whether a suggestion was helpful or not.
Building a Robust Search System Beyond Autocomplete
Creating an effective autocomplete feature is more than just combining JavaScript, HTML, and CSS. To offer a robust search experience, you need to access the right data sources and have a backend that can efficiently deliver results to the front end for user display. Additionally, there are numerous possibilities for enhancements as you delve deeper into what’s achievable.
Here are a couple of noteworthy considerations when it comes to search suggestions:
Fuzzy Search for Spelling Mistakes: Autocomplete should be able to intelligently handle misspellings. Even when a user misspells a query like “iPhone,” the system should suggest relevant results based on what the user likely intended. Implementing fuzzy searching techniques can help achieve this. However, it’s crucial to ensure that the implementation of fuzzy search doesn’t negatively impact performance.
Category-Based Search: Some queries may benefit from category-based filtering, allowing users to drill down into more specific search results within certain categories. This advanced feature requires additional data structuring within your search database. Users can refine their search results based on attributes or categories by applying filters to the data for a faceted search.
To facilitate typo tolerance and category-based searching, you may need to tailor your database structure and search algorithms accordingly.
For a comprehensive and robust search solution, you might consider exploring specialized tools and platforms like PartsLogic, which offer built-in support for search suggestions and faceted search capabilities through APIs. Such platforms can provide a solid foundation for creating advanced and user-friendly search experiences, saving you the effort of building these features from scratch.
In summary, building a robust search system goes beyond simple autocomplete and involves considering factors like typo tolerance, category-based filtering, and data organization. Leveraging specialized platforms and tools can streamline the development process and ensure a highly effective search experience for your users.