Search algorithms are fundamental to computer science, enabling efficient data retrieval in various applications, from web search engines to database management systems. This blog will explore the types of search algorithms, their applications, and how to determine the best search algorithm for specific tasks.
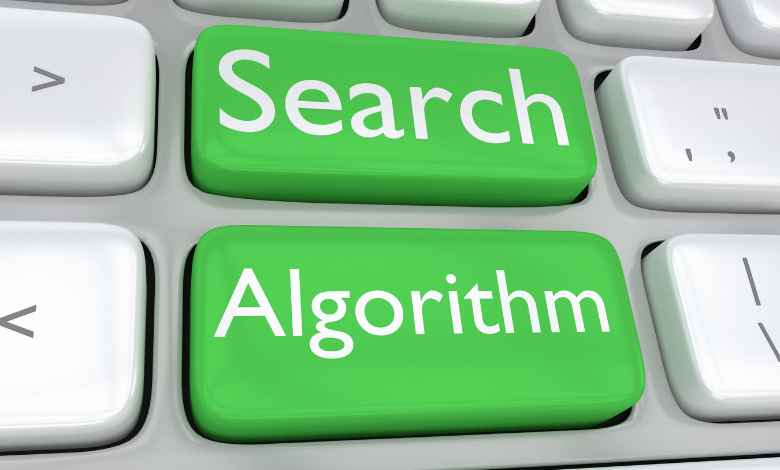
Introduction to Search Algorithms
A search algorithm is a systematic process designed to find specific information within a larger dataset. These algorithms are essential in solving search problems and are categorized based on their structure, efficiency, and the type of data they handle. Understanding the types of search algorithms can help in selecting the best search algorithm for a given problem.
Choosing the optimal search algorithm hinges on the particular use case, the dataset’s size, and whether the data is sorted. Here is a summary to help choose the best search algorithm:
- Linear Search: Best for small, unsorted datasets.
- Binary Search: Best for large, sorted datasets.
- Depth-First Search (DFS): Best for deep tree or graph structures.
- Breadth-First Search (BFS): Ideal for finding the shortest path in unweighted graphs.
- Interpolation Search: Best for uniformly distributed, sorted datasets.
- Exponential Search: Best for large, sorted datasets with unknown ranges.
- Jump Search: Best for sorted arrays with large datasets.
- Fibonacci Search: Best for large, sorted datasets using Fibonacci number properties.
Linear Search
Definition and Explanation
Linear search, also known as sequential search, is the most straightforward search algorithm. It works by checking each element in the list one by one, starting from the beginning, until it either finds the desired element or reaches the end of the list.
Algorithm
def linear_search(arr, target):
for i in range(len(arr)):
if arr[i] == target:
return i
return -1
Use Cases
- Small datasets
- Unsorted lists
- Simple implementation
Advantages
- Easy to implement
- No need for sorted data
Disadvantages
- Inefficient for large datasets (O(n) time complexity)
Binary Search
Definition and Explanation
Binary search is a highly efficient algorithm used to locate an item within a sorted list. The process involves repeatedly dividing the search interval by half until the desired item is found or the interval is empty.
Algorithm
def binary_search(arr, target):
left, right = 0, len(arr) – 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid – 1
return -1
Use Cases
- Large datasets
- Sorted lists
Advantages
- More efficient than linear search (O(log n) time complexity)
- Suitable for large datasets
Disadvantages
- Requires sorted data
- More complex implementation
Depth-First Search (DFS)
Definition and Explanation
Depth-First Search (DFS) is a fundamental algorithm used for traversing or searching tree or graph data structures. This recursive algorithm begins at the root node and delves deep into each branch before retracing its steps. By exploring as far as possible along a branch before backtracking, DFS effectively navigates through all the nodes in a systematic manner.
Algorithm
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
for next in graph[start] – visited:
dfs(graph, next, visited)
return visited
Use Cases
- Solving puzzles
- Pathfinding in games
- Graph traversal
Advantages
- Low memory usage (O(V) where V is the number of vertices)
- Can be implemented using a stack to avoid recursion
Disadvantages
- Can get stuck in deep paths if the graph is vast
- Not guaranteed to find the shortest path
Breadth-First Search (BFS)
Definition and Explanation
Breadth-First Search (BFS) is an algorithm used to traverse or search tree or graph data structures. It begins at the root node and explores all neighboring nodes at the current depth before progressing to nodes at the next depth level.
Algorithm
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
while queue:
vertex = queue.popleft()
if vertex not in visited:
visited.add(vertex)
queue.extend(graph[vertex] – visited)
return visited
Use Cases
- Finding the shortest path in an unweighted graph
- Web crawlers
- Network broadcasting
Advantages
- Guaranteed to find the shortest path in unweighted graphs
- Simple implementation
Disadvantages
- High memory usage (O(V) where V is the number of vertices)
- Can be slower for deep graphs
Interpolation Search
Definition and Explanation
Interpolation search is an algorithm designed to search for a specific key in an array sorted by numerical key values. It enhances the traditional binary search by considering the distribution of the data within the array, allowing for potentially faster site search times when the data is uniformly distributed.
Algorithm
def interpolation_search(arr, target):
low = 0
high = len(arr) – 1
while low <= high and arr[low] <= target <= arr[high]:
pos = low + ((target – arr[low]) * (high – low) // (arr[high] – arr[low]))
if arr[pos] == target:
return pos
if arr[pos] < target:
low = pos + 1
else:
high = pos – 1
return -1
Use Cases
- Uniformly distributed datasets
Advantages
- Faster than binary search for uniformly distributed data (O(log log n) time complexity)
Disadvantages
- Requires uniformly distributed data
- More complex than a binary search
Exponential Search
Definition and Explanation Exponential search is an algorithm that finds the range where the target value lies and then performs a binary search within that range.
Algorithm
def exponential_search(arr, target):
if arr[0] == target:
return 0
index = 1
while index < len(arr) and arr[index] <= target:
index = index * 2
return binary_search(arr[:min(index, len(arr))], target)
Use Cases
- Large datasets
- Sorted arrays
Advantages
- Efficient for large datasets (O(log n) time complexity)
- Useful when the range of the search is unknown
Disadvantages
- Requires sorted data
- The initial exponential phase can be slow for large gaps
Jump Search
Definition and Explanation
Jump search is an algorithm used to find a key in a sorted array. It works by jumping ahead by a fixed number of steps to quickly narrow down the search region and then performing a linear search within the identified block.
Algorithm
import math
def jump_search(arr, target):
n = len(arr)
step = int(math.sqrt(n))
prev = 0
while arr[min(step, n) – 1] < target:
prev = step
step += int(math.sqrt(n))
if prev >= n:
return -1
for i in range(prev, min(step, n)):
if arr[i] == target:
return i
return -1
Use Cases
- Sorted arrays
- Large datasets
Advantages
- More efficient than linear search (O(√n) time complexity)
- Simpler than a binary search for an implementation
Disadvantages
- Requires sorted data
- Less efficient than binary search for very large datasets
Fibonacci Search
Definition and Explanation Fibonacci search is a search algorithm that uses Fibonacci numbers to divide the array into sub-arrays, making it similar to binary search but with slightly different partitioning.
Algorithm
def fibonacci_search(arr, target):
fib_m2 = 0
fib_m1 = 1
fib_m = fib_m1 + fib_m2
n = len(arr)
while fib_m < n:
fib_m2 = fib_m1
fib_m1 = fib_m
fib_m = fib_m1 + fib_m2
offset = -1
while fib_m > 1:
i = min(offset + fib_m2, n – 1)
if arr[i] < target:
fib_m = fib_m1
fib_m1 = fib_m2
fib_m2 = fib_m – fib_m1
offset = i
elif arr[i] > target:
fib_m = fib_m2
fib_m1 = fib_m1 – fib_m2
fib_m2 = fib_m – fib_m1
else:
return i
if fib_m1 and arr[offset + 1] == target:
return offset + 1
return -1
Use Cases
- Large datasets
- Sorted arrays
Advantages
- Uses the mathematical properties of Fibonacci numbers
- Efficient for large datasets (O(log n) time complexity)
Disadvantages
- Requires sorted data
- More complex than a binary search
Conclusion
Choosing the most suitable search algorithm depends on the specific requirements of the use case, the size of the dataset, and whether the data is already sorted. Each type of searching algorithm has its strengths and weaknesses. The choice of the best search algorithm will depend on the context and requirements of the specific problem at hand.
If you are looking for a comprehensive solution that can help integrate these search algorithms into your applications effectively, consider using PartsLogic. PartsLogic offers advanced algorithms and tools that can enhance your search capabilities, ensuring efficient and accurate data retrieval tailored to your needs.